Lesson 3. Introduction to Working With JSON Data in Open Source Python
Learning Objectives
After completing this tutorial, you will be able to:
- Create and convert
JSON
objects usingPython
.
What You Need
You will need a computer with internet access to complete this lesson.
In this lesson, you will explore the machine readable JSON data structure. Machine readable data structures are more efficient - particularly for larger data that contain hierarchical structures.
Review JSON
Recall from the previous lesson that the structure of a JSON
object is as follows:
- The data are in name/value pairs using colons
:
. - Data objects are separated by commas.
- Curly braces
{}
hold the objects. - Square brackets
[]
can be used to indicate an array that contains a group of objects. - Each data element is enclosed with quotes
""
if it is a character, or without quotes if it is a numeric value.
Example:
{ "name":"Chaya", "age":12, "city":"Boulder", "type":"Canine" }
You also learned that the Python
library json
is helpful to convert data from lists or dictonaries into JSON
strings and JSON
strings into lists or dictonaries. Pandas
can also be used to convert JSON
data (via a Python
dictionary) into a Pandas
DataFrame
.
In this lesson, you will use the json
and Pandas
libraries to create and convert JSON
objects.
Work with JSON Data in Python
Python Dictionary to JSON
Using the Python
json
library, you can convert a Python
dictionary to a JSON
string using the json.dumps()
function.
Begin by creating the Python
dictionary that will be converted to JSON
.
import json
import pandas as pd
# Create and populate the dictionary
dict = {}
dict["name"] = "Chaya"
dict["age"] = 12
dict["city"] = "Boulder"
dict["type"] = "Canine"
dict
{'name': 'Chaya', 'age': 12, 'city': 'Boulder', 'type': 'Canine'}
Notice below that the Python
dictionary and the JSON
string look very similiar, but that the JSON
string is enclosed with quotes ''
.
json_example = json.dumps(dict, ensure_ascii=False)
json_example
'{"name": "Chaya", "age": 12, "city": "Boulder", "type": "Canine"}'
Recall that you use type()
to check the object type, and notice that the JSON
is of type str
.
type(json_example)
str
JSON to Python Dictionary
You can also manually define JSON
by enclosing the JSON
with quotes ''
.
json_sample = '{ "name":"Chaya", "age":12, "city":"Boulder", "type":"Canine" }'
type(json_sample)
str
Using the json.loads()
function, a JSON
string can be converted to a dictionary
.
# Load JSON into dictionary
data_sample = json.loads(json_sample)
data_sample
{'name': 'Chaya', 'age': 12, 'city': 'Boulder', 'type': 'Canine'}
You can check the type again to see that it has been converted to a Python
dictionary.
type(data_sample)
dict
Recall that you can call any key of a Python
dictionary and see the associated values.
data_sample["name"]
'Chaya'
data_sample["city"]
'Boulder'
Python Dictionary to Pandas Dataframe
If desired, you can use the from_dict()
function from Pandas
to read the dictionary into a Pandas
Dataframe
.
df = pd.DataFrame.from_dict(data_sample, orient='index')
df
0 | |
---|---|
name | Chaya |
age | 12 |
city | Boulder |
type | Canine |
Pandas Dataframe to JSON
Conversely, you can also convert a Pandas
Dataframe
to JSON
using the Pandas
method to_json()
.
sample_json = df.to_json(orient='split')
type(sample_json)
str
You now know the basics of creating and converting JSON
objects using Python
. In the next lessons, you will work with hierarchical JSON
data accessed via RESTful APIs.
Share on
Twitter Facebook Google+ LinkedIn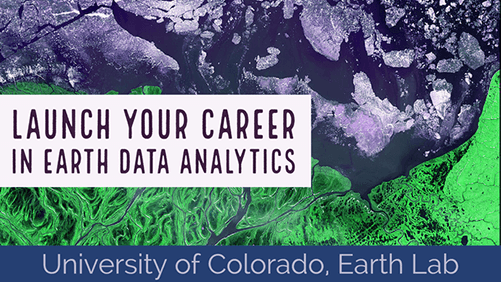
Leave a Comment